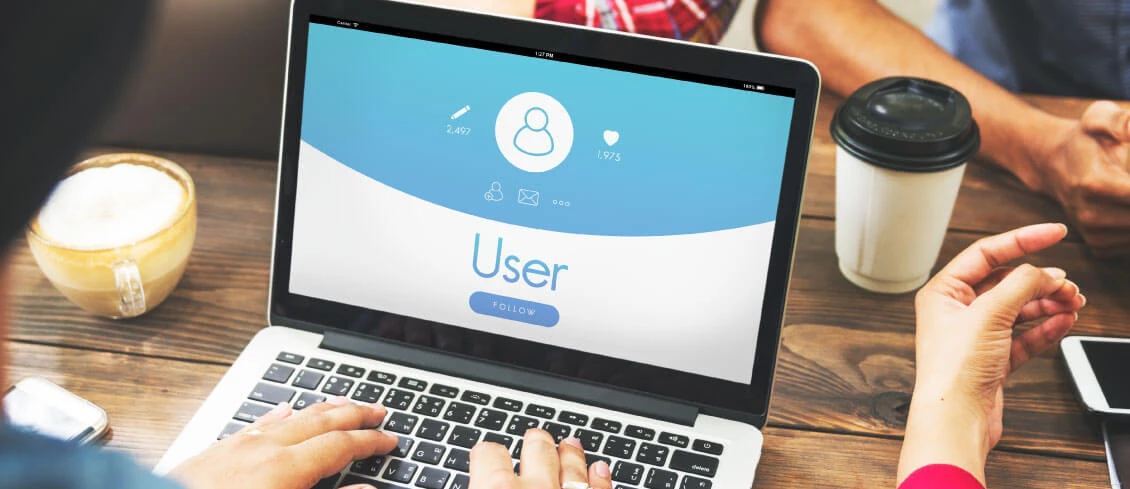
- Views: 5.0K
- Category: Codeigniter
- Published at: 13 Apr, 2020
- Updated at: 12 Aug, 2023
Registration OR Signup system in Codeigniter 4 with Login
The registration system on any website is the backbone, and every system has its registration system according to need. We will create the Registration/Signup system in Codeigniter 4 with Login.
Some third-party libraries/auth system in Codeigniter 4 is also available to embed that they have the beta version; that's why they are not considering the auth.
We will create the registration system in Codeigniter from scratch; you can easily understand how to do it.
Step 1: Download the Codeigniter 4 and configure the base_url. So go to the folder app/config/app.php and open it, set the base_url
public $baseURL = 'http://localhost/ci4signup/';
Step 2: Create a database named "ci4signup" and create the table.
CREATE TABLE `users` (
`u_id` int(11) NOT NULL,
`u_name` varchar(200) NOT NULL,
`u_email` varchar(250) NOT NULL,
`u_password` varchar(250) NOT NULL,
`u_link` varchar(250) NOT NULL,
`u_date` datetime NOT NULL,
`u_updated` datetime DEFAULT NULL,
`u_status` int(11) DEFAULT '0'
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
ALTER TABLE `users`
ADD PRIMARY KEY (`u_id`);
ALTER TABLE `users`
MODIFY `u_id` int(11) NOT NULL AUTO_INCREMENT;
COMMIT;
Step 3: Open your database.php file by going to app/config/database.php and connect the database in Codeigniter 4
public $default = [
'DSN' => '',
'hostname' => 'localhost',
'username' => 'root',
'password' => '',
'database' => 'ci4signup',
'DBDriver' => 'MySQLi',
'DBPrefix' => '',
'pConnect' => false,
'DBDebug' => (ENVIRONMENT !== 'production'),
'cacheOn' => false,
'cacheDir' => '',
'charset' => 'utf8',
'DBCollat' => 'utf8_general_ci',
'swapPre' => '',
'encrypt' => false,
'compress' => false,
'strictOn' => false,
'failover' => [],
'port' => 3306,
];
Step 4: Create a controller named User and use the code below.
<?php
namespace App\Controllers;
use App\Models\ModUsers;
class User extends BaseController
{
public function index()
{
echo 'user is wroking..';
}
public function register()
{
helper('form');
$session = \Config\Services::session();
$data['message'] = $session->getFlashdata('message');
echo view('signup',$data);
}
public function newuser()
{
$myvalues = $this->validate(
[
'name'=>'required',
'email'=>'required',
'password'=>'required',
]
);
if (!$myvalues) {
$this->register();
}
else{
$myrequest = \Config\Services::request();
$session = \Config\Services::session();
$users = new ModUsers();
helper('text');
$data['u_name'] = $myrequest->getPost('name');
$data['u_email'] = $myrequest->getPost('email');
$data['u_password'] = $myrequest->getPost('password');
$data['u_password'] = hash('md5',$data['u_password']);
$data['u_link'] = random_string('alnum',20);
$message = "Please activate the account ".anchor('user/activate/'.$data['u_link'],'Activate Now','');
$checkAlreadyUser = $users->where('u_email',$data['u_email'])->findAll();
if (count($checkAlreadyUser) > 0) {
$session->setFlashdata('message','This email: ' . $data['u_email'] . ' already exist.');
return redirect()->to(site_url('user/newuser'));
//echo 'the email is already exist.';
}
else{
$myNewUser = $users->insert($data);
if ($myNewUser) {
$email = \Config\Services::email();
$email->setFrom('ci4signup@shekztech.com', 'Activate the account');
$email->setTo($data['u_email']);
$email->setSubject('Activate the account | shekztech.com');
$email->setMessage($message);
$email->send();
$email->printDebugger(['headers']);
}
else{
$session->setFlashdata('message','We have successfully create the account but we can\'t send you the email right now.');
return redirect()->to(site_url('user/newuser'));
}
}
//die();
}
}
public function activate($linkHere)
{
//echo $linkHere;
$session = \Config\Services::session();
$user = new ModUsers();
$checkUserLink = $user->where('u_link',$linkHere)->findAll();
if (count($checkUserLink) > 0) {
$data['u_status'] = 1;
$data['u_link'] = $checkUserLink[0]['u_link'].'ok';
$activateUser = $user->update($checkUserLink[0]['u_id'],$data);
if ($activateUser) {
$session->setFlashdata('message','We have successfully activate your account.');
return redirect()->to(site_url('user/newuser'));
}
else{
$session->setFlashdata('message','Your link is not available in the system, please check your email address and try again.');
return redirect()->to(site_url('user/newuser'));
}
}
else{
$session->setFlashdata('message','Something went wrong.');
return redirect()->to(site_url('user/newuser'));
}
// var_dump($checkUserLink);
}
public function signin()
{
$session = \Config\Services::session();
$data['message'] = $session->getFlashdata('message');
helper('form');
echo view('signin',$data);
}
public function checkuser()
{
$myrequest = \Config\Services::request();
$session = \Config\Services::session();
$myvalues = $this->validate(
[
'email'=>'required',
'password'=>'required',
]
);
if (!$myvalues) {
$this->signin();
}
else{
$users = new ModUsers();
helper('text');
$data['u_email'] = $myrequest->getPost('email');
$data['u_password'] = $myrequest->getPost('password');
$data['u_password'] = hash('md5',$data['u_password']);
$allUsers = $users->where('u_email',$data['u_email'])->findAll();
if (count($allUsers) > 0) {
if ($data['u_password'] == $allUsers[0]['u_password']) {
$sessionData['u_id'] = $allUsers[0]['u_id'];
$sessionData['u_name'] = $allUsers[0]['u_name'];
$sessionData['u_email'] = $allUsers[0]['u_email'];
$sessionData['u_date'] = $allUsers[0]['u_date'];
$sessionData['u_updated'] = $allUsers[0]['u_updated'];
$sessionData['u_status'] = $allUsers[0]['u_status'];
$session->set($sessionData);
if ($session->get('u_id')) {
return redirect()->to(site_url('home'));
}
else{
$session->setFlashdata('message','You can\'t signin, please try again.');
return redirect()->to(site_url('user/signin'));
}
}
else{
$session->setFlashdata('message','The password is invalid, please check your password.');
return redirect()->to(site_url('user/signin'));
}
}else{
$session->setFlashdata('message','The user is not available in the system.');
return redirect()->to(site_url('user/signin'));
}
}
}
public function signout()
{
$session = \Config\Services::session();
$session->destroy();
return redirect()->to(site_url('user/signin'));
}
}//class here
Step 4: Create a model named ModUsers and use the code below.
<?php
namespace App\Models;
use CodeIgniter\Model;
class ModUsers extends Model
{
protected $DBGroup = 'default';
protected $table = 'users';
protected $primaryKey = 'u_id';
protected $returnType = 'array';
protected $useTimestamps = true;
protected $allowedFields = ['u_name','u_email','u_password','u_link','u_status'];
protected $createdField = 'u_date';
protected $updatedField = 'u_updated';
}
Step 5: This is an email configuration file in Codeigniter 4 to send the email from the localhost environment.
<?php
namespace Config;
use CodeIgniter\Config\BaseConfig;
class Email extends BaseConfig
{
public $fromEmail;
public $fromName;
public $recipients;
public $userAgent = 'CodeIgniter';
public $protocol = 'smtp';
public $mailPath = '/usr/sbin/sendmail';
public $SMTPHost='mail.shekztech.com';
public $SMTPUser = 'ci4signup@shekztech.com';
public $SMTPPass = 'YourPasswordHere';
public $SMTPPort = 587;
public $SMTPTimeout = 15;
public $SMTPKeepAlive = false;
public $SMTPCrypto = 'tls';
public $wordWrap = true;
public $wrapChars = 76;
public $mailType = 'html';
public $charset = 'UTF-8';
public $validate = false;
public $priority = 3;
public $CRLF = "\r\n";
public $newline = "\r\n";
public $BCCBatchMode = false;
public $BCCBatchSize = 200;
public $DSN = false;
}
Step 6: Create a view named signup and use the code below.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Register a New </title>
</head>
<body>
<?php if (isset($message) && !empty($message)):
echo $message;
?>
<?php endif; ?>
<?php echo \Config\Services::validation()->listErrors()?>
<?php echo form_open('user/newuser'); ?>
<p>
Enter your name <?php echo form_input('name','',''); ?>
</p>
<p>
Enter your email <?php echo form_input('email','',''); ?>
</p>
<p>
Enter your password <?php echo form_password('password','',''); ?>
</p>
<p>
<?php echo form_submit('mybutton','Create Now',''); ?>
</p>
<?php form_close(); ?>
</body>
</html>
Step 7: Create another view for the sign-in form.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Register a New </title>
</head>
<body>
<?php if (isset($message) && !empty($message)):
echo $message;
?>
<?php endif; ?>
<?php echo \Config\Services::validation()->listErrors()?>
<?php echo form_open('user/checkuser'); ?>
<p>
Enter your email <?php echo form_input('email','',''); ?>
</p>
<p>
Enter your password <?php echo form_password('password','',''); ?>
</p>
<p>
<?php echo form_submit('mybutton','Login Now',''); ?>
</p>
<?php form_close(); ?>
</body>
</html>
Step 8: Create a controller named home.
<?php namespace App\Controllers;
class Home extends BaseController
{
public function index()
{
$session = \Config\Services::session();
$checkUser = $session->get('u_id');
//return view('welcome_message');
if ($checkUser) {
echo 'welcome: ' . $session->get('u_name');
}
else{
echo 'redirect here...';
}
}
}
Download the source code
0 Comment(s)