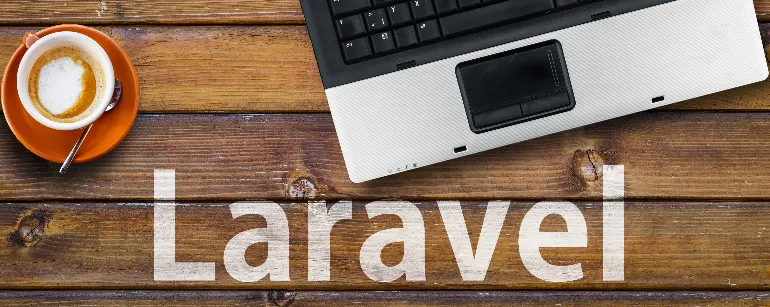
- Views: 2.7K
- Category: Laravel
- Published at: 31 Aug, 2023
- Updated at: 01 Sep, 2023
Creating and Managing Database Seeding in Laravel 10 with Seeders
Introduction
Database seeding is a powerful feature in Laravel that allows developers to populate tables with sample data. Laravel 10 enhances this feature, providing a streamlined way to manage seeders and factories. Whether you are new to Laravel or looking to upgrade your knowledge with the latest version, this tutorial will walk you through setting up and running database seeders for a Category model.
Step 1: Generating Files with Artisan
Firstly, create the necessary model, migration, and factory files using Laravel's Artisan command-line tool. Open your terminal and run the following command:
php artisan make:model Category -mf
This command performs three tasks:
It generates a Category model in the app/Models directory.
It creates a migration file in the database/migrations directory.
It produces a factory file in the database/factories directory.
Step 2: Defining the Category Model
Navigate to app/Models/Category.php. Here, we define the attributes that our Category model will have.
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
class Category extends Model
{
use HasFactory, SoftDeletes;
protected $fillable = ['name', 'slug', 'user_id'];
...
}
Note the use of the SoftDeletes trait. This allows us to 'softly' delete records without removing them from the database.
Step 3: Crafting the Migration
Migrations serve as version control for your database, allowing you to modify your database structure in a structured and organized way. Your migration file will be located at database/migrations/date_create_categories_table.php.
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateCategoriesTable extends Migration
{
public function up(): void
{
Schema::create('categories', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('slug')->index();
$table->foreignId('user_id')->constrained('users')->onDelete('cascade');
$table->timestamps();
$table->softDeletes();
});
}
public function down(): void
{
Schema::dropIfExists('categories');
}
}
To run this migration, you can use:
php artisan migrate
Step 4: Creating the Factory
Factories are used to generate data for your models. Open the CategoryFactory.php located at database/factories/CategoryFactory.php.
namespace Database\Factories;
use App\Models\User;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
class CategoryFactory extends Factory
{
public function definition(): array
{
$name = $this->faker->words(2, true);
return [
'name' => $name,
'slug' => Str::slug($name),
'user_id' => User::factory()
];
}
}
Step 5: Creating the Seeder
Now we'll create a seeder to populate our categories table. Use the following Artisan command to generate a new seeder:
php artisan make:seeder CategoriesTableSeeder
Open the generated CategoriesTableSeeder.php file located at database/seeders/.
namespace Database\Seeders;
use App\Models\Category;
use Illuminate\Database\Seeder;
class CategoriesTableSeeder extends Seeder
{
public function run(): void
{
Category::factory()
->times(50)
->create();
}
}
Step 6: Register the Seeder in DatabaseSeeder
Finally, we need to register our new seeder in DatabaseSeeder.php so that it runs when we seed the database.
namespace Database\Seeders;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
public function run(): void
{
$this->call([
CategoriesTableSeeder::class,
]);
}
}
To run the seeder, execute the following command:
php artisan db:seed
And there you have it! You've now set up a comprehensive database seeding system in Laravel 10. You can now seed your categories table with 50 sample records, each associated with a user.
Conclusion
Mastering database seeding in Laravel 10 is more than just about writing code; it's about understanding the fundamentals that make this framework so robust and developer-friendly. From utilizing Artisan commands that scaffold much of your boilerplate code to understanding how Eloquent ORM works hand-in-hand with factories and seeders, every step is an important cog in the Laravel ecosystem. With these tools, you can create scalable, maintainable, and testable applications.
This tutorial aimed to guide you through the intricacies of setting up a database seeder, and using it in conjunction with factories and migrations. By now, you should have a solid grasp of these core concepts, enabling you to efficiently generate realistic test data for your application's database tables. Armed with this knowledge, you're one step closer to becoming a Laravel expert, capable of tackling a broader range of challenges in your future projects.
So, go ahead take this knowledge, and build something amazing. No matter your experience level as a developer, never stop learning, because the landscape of web development is always evolving. We appreciate your time in reading this blog, and if you have any questions, insights, or feedback, feel free to leave them in the comments section below. Happy coding!
0 Comment(s)