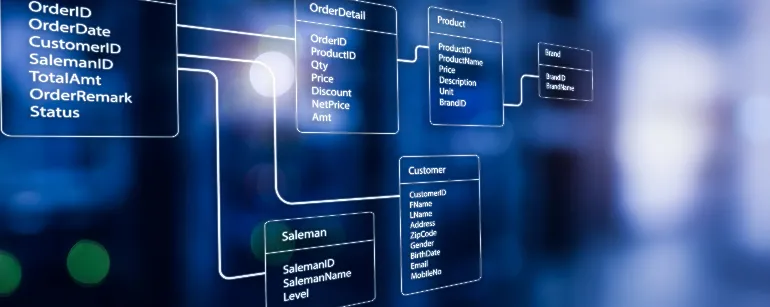
- Views: 1.3K
- Category: Laravel
- Published at: 03 Sep, 2023
- Updated at: 07 Sep, 2023
How to use the trasaction in Codeigniter 4
In the realm of web development, database transactions play a pivotal role in ensuring data integrity. Imagine you're building an application involving a fund transfer between two user accounts. If one part of the transaction (e.g., deducting money from Account A) is successful but the other (adding money to Account B) fails, your database will be left in an inconsistent state. CodeIgniter 4 provides a built-in mechanism to handle transactions efficiently. Utilizing the transaction methods, you can ensure that multiple database operations either all succeed or all fail together, maintaining database integrity.
Transactions in CodeIgniter 4 are simple yet powerful. The framework offers various transaction methods like transStart()
, transComplete()
, transStatus()
, transCommit()
, and transRollback()
that make it easier to implement transactions. The transStart()
initiates a new database transaction and turns off auto-commit. The transComplete()
will automatically determine if the transaction should be committed or rolled back. transStatus()
checks if the transaction was successful. If something goes wrong, transRollback()
is used to undo all database work done during the current transaction, ensuring that your database remains consistent.
Example Implementation
Routes
First, let's define a route in your Routes.php
file.
$routes->add('transfer', 'UserController::sendUserFundTransfer');
Controller
Create a UserController.php
with the following code.
<?php
namespace App\Controllers;
use App\Models\ModUserCash;
class UserController extends BaseController
{
public function sendUserFundTransfer($cash, $userWithdrawCurrent, $withdID)
{
$tableModUserCash = new ModUserCash();
$this->db->transStart();
// Update the withdrawal ID with current withdrawal status
$this->update($withdID, $userWithdrawCurrent);
// Insert cash into user cash box
$tableModUserCash->insert($cash);
$this->db->transComplete();
if ($this->db->transStatus() === FALSE)
{
$this->db->transRollback();
return false;
}
else{
$this->db->transCommit();
return true;
}
}
private function update($withdID, $userWithdrawCurrent)
{
// Update logic here
}
}
Model
Create ModUserCash.php
for the model.
<?php
namespace App\Models;
use CodeIgniter\Model;
class ModUserCash extends Model
{
protected $table = 'user_cash';
// Insert, Update, Delete methods go here
}
Conclusion
Understanding how to implement transactions in CodeIgniter 4 can significantly improve the robustness of your application. It allows you to execute multiple database operations in a safe and atomic way. If any part of the transaction fails, all changes are rolled back, and your database remains in a consistent state. This makes CodeIgniter 4 an excellent choice for building reliable, robust web applications that handle critical operations like fund transfers.
0 Comment(s)