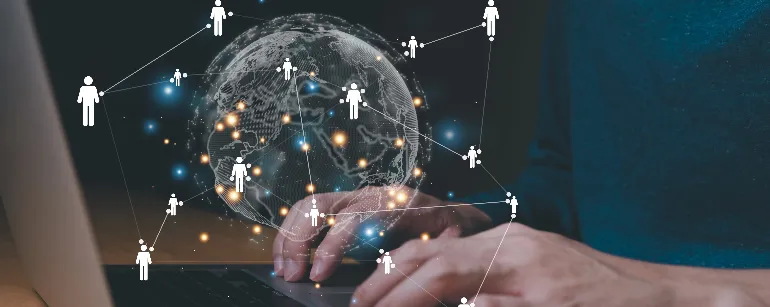
- Views: 498
- Category: Laravel
- Published at: 01 Sep, 2023
- Updated at: 01 Sep, 2023
How to Create Model and use Eloquent ORM in laravel 10
As Laravel continues to evolve, its ecosystem brings new features that make development faster, more efficient, and more enjoyable. Laravel 10 is no exception and offers a plethora of tools for developers to harness. One such tool is Eloquent ORM (Object-Relational Mapping), an elegant way of interacting with your database using object-oriented syntax. Eloquent makes it extremely easy to perform CRUD (Create, Read, Update, Delete) operations and also map relationships between different database tables.
In this blog post, we're going to focus on creating a new model in Laravel 10 and utilizing Eloquent ORM to interact with our database. By taking the example of CourseCategory—a model that represents categories for different courses—we'll learn how to set up a migration, define or make a model, and explore Eloquent relationships. If you've been looking for a guide that breaks down these topics into bite-sized, understandable pieces, you're in the right place.
Creating Migration and Model via Artisan Command
To start off, we'll create a new migration and model using Laravel's Artisan command-line tool. Artisan makes this process a breeze. For our CourseCategory model and corresponding migration, you can execute the following command:
php artisan make: model CourseCategory -m
The -m flag will also be Alongside the model, you need to create a migration file. The migration will reside in your database/migrations directory. For our example, the migration might look something like this:
Migration: CreateCourseCategoriesTable.php
Your migration file would be typically located in the database/migrations/ directory. Here's the content:
public function up(): void
{
Schema::create('course_categories', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('user_id');
$table->string('name');
$table->string('slug')->index();
$table->softDeletes();
$table->timestamps();
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('course_categories');
}
Model: CourseCategory.php
Your model would generally be in the app/Models/ directory. Below is your model file.
<?php
class CourseCategory extends Model
{
use HasFactory, SoftDeletes;
protected $fillable = ['name', 'slug', 'user_id'];
public function user() {
return $this->belongsTo(User::class);
}
public function courses() {
return $this->hasMany(Course::class);
}
public function courseSubCategories() {
return $this->hasMany(CourseSubCategory::class);
}
}
Now, when you have finished configuring this migration and model, you need to start the migration by running the following command, which will create the course_categories table in your database:
php artisan migrate for PHP.
And there you have it! You've now created a migration and model in Laravel 10 that uses Eloquent ORM for data manipulation. You can now use Eloquent methods to handle database operations related to the CourseCategory model.
Using Eloquent ORM in the Model
After creating the migration and model, let's add some functionality to our CourseCategory model. I see you've already set up the model to use the SoftDeletes trait and defined relationships with User, Course, and CourseSubCategory models. Let's explore how to use these relationships in Eloquent.
To retrieve the user associated with a particular course category, you would do:
$courseCategory = CourseCategory::find(1); // 1 is the ID of the course category
$user = $courseCategory->user; // This will return the User model that owns this course category
To fetch all courses under a particular category:
$courses = $courseCategory->courses; // Returns a collection of Course models
To fetch all sub-categories under a particular course category:
$courseSubCategories = $courseCategory->courseSubCategories; // Returns a collection of CourseSubCategory models
And there you have it! With Eloquent ORM, handling database operations and relationships is incredibly straightforward. Stay tuned as we delve deeper into Eloquent's more advanced features in the upcoming sections.
By using Laravel 10 and Eloquent, you can make your back-end logic as eloquent as your application's user interface.
Conclusion
In this blog post, we've explored how to create a model and migration in Laravel 10 using Artisan commands. We focused on the CourseCategory example to demonstrate the power of Eloquent ORM for efficient database interactions. With these tools, managing your database becomes a straightforward, clean process.
0 Comment(s)