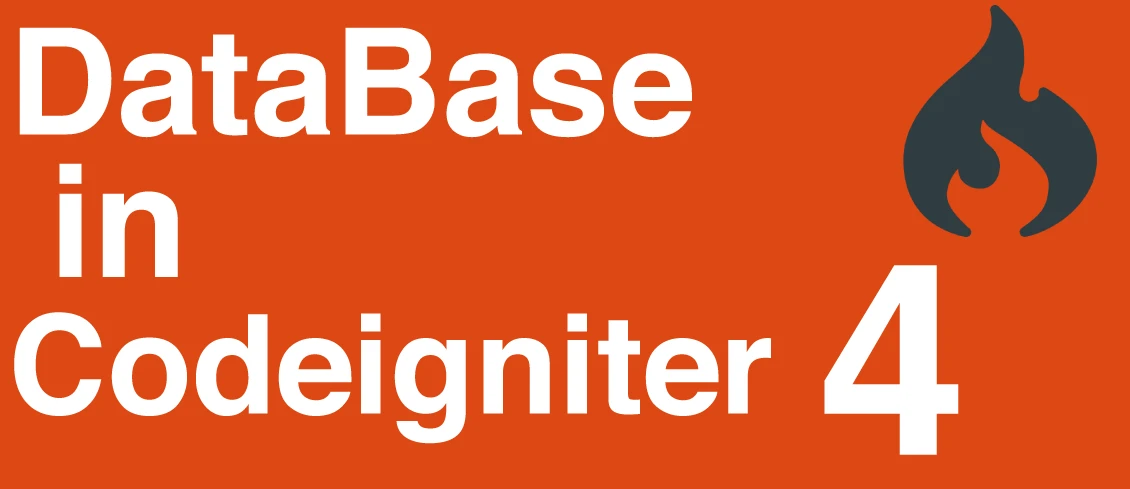
- Views: 6.0K
- Category: Codeigniter
- Updated at: 19 Aug, 2023
How to connect database in Codeigniter 4
How to connect the database in Codeigniter 4
Connecting your database with your Codeigniter 4 application is different from Codeigniter 3. In Codeigniter 3, we always connect our database by adding the username, password, and database name. That's it, but in CodeIgniter 4, you must take extra steps to connect the database with our application.
Step 1: Download the Codeigniter 4 and configure the base_url; in my case, I am using this base_url='http://localhost/crudci4/public/' for base_url; go to the folder app/config/app.php
Step 2: Create a database named "crudci4".
Step 3: Create the student table while working on data modeling in Codeigniter 4.
create table students (
s_id int auto_increment primary key,
s_name varchar(200) not null,
s_date datetime not null,
s_subject varchar(200) not null,
s_update datetime null
);
Step 4: Go to the folder (app/config) and open the database.php file.
Step 5: If you are locally connected, set username root and password '', but if you have connected with your server, use your username=>'YourUserName' and password=>'YourPassword,' and finally set your default group.
Step 6: Create the controller named "Students" Copy the below code and paste the code into the file, which you have just created.
<?php
namespace App\Controllers;
use App\Models\MyStudents;
use CodeIgniter\Config\Config;
use CodeIgniter\Controller;
class Students extends Controller
{
public function index()
{
$session = \Config\Services::session();
$message = $session->getFlashdata('message');
$std = new MyStudents();
$data['students'] = $std->findAll();
$pager = \Config\Services::pager();
$data['message'] = $message;
echo view('students',$data);
//var_dump($results);
//echo 'index Students';
}
public function newstudent()
{
echo view('newstudent');
//echo ' new method';
}
public function addstudent()
{
$request = \Config\Services::request();
$session = \Config\Services::session();
$name = $request->getPost('std');
$subject = $request->getPost('subject');
$newStudent = [
's_name'=>$name,
's_subject'=>$subject,
];
$std = new MyStudents();
$result = $std->insert($newStudent);
if ($result) {
$session->setFlashdata('message','You have successfully inserted the student.');
}
else{
$session->setFlashdata('message','Oops something went wrong please try again.');
}
return redirect()->to(site_url('students'));
//var_dump($result);
//echo 'working..';
}
public function editstudent($userId = null)
{
$session = \Config\Services::session();
if (!empty($userId)) {
$std = new MyStudents();
$result = $std->where('s_id',$userId)->findAll();
if (count($result) > 0) {
$data['student'] = $result;
echo view('editStudent',$data);
}
else{
$session->setFlashdata('message','The Student is not exist');
return redirect()->to(site_url('students'));
}
}
else{
$session->setFlashdata('message','The id is not available, please try again.');
return redirect()->to(site_url('students'));
}
}
public function updatestudent()
{
$request = \Config\Services::request();
$session = \Config\Services::session();
$name = $request->getPost('std');
$subject = $request->getPost('subject');
$studentId = $request->getPost('id');
$updateStudent = [
's_name'=>$name,
's_subject'=>$subject,
];
//echo $studentId;
//die();
$std = new MyStudents();
$result = $std->update($studentId,$updateStudent);
if ($result) {
$session->setFlashdata('message','You have successfully updated the student.');
}
else{
$session->setFlashdata('message','Oops something went wrong please try again.');
}
return redirect()->to(site_url('students'));
}
public function delete($userId)
{
$session = \Config\Services::session();
if (!empty($userId)) {
$std = new MyStudents();
$result = $std->where('s_id',$userId)->findAll();
if (count($result) > 0) {
//$result = $std->delete($userId);
$result = $std->where('s_id',$userId)->delete();
if ($result){
$session->setFlashdata('message','You have successfully deleted.');
return redirect()->to(site_url('students'));
}
else{
$session->setFlashdata('message','You can\'t delete the student right now.');
return redirect()->to(site_url('students'));
}
}
else{
$session->setFlashdata('message','The Student is not exist');
return redirect()->to(site_url('students'));
}
}
else{
$session->setFlashdata('message','The id is not available, please try again.');
return redirect()->to(site_url('students'));
}
}
}//class
Step 7: Create a model named "MyStudents" and keep it in your model's folder; copy the code below and paste it into your model, which you have just created.
Step 8: Go to the folder(app/config) and open the files named Routes.php find the comment "Route Definitions" and paste this code $routes->get('/', 'Students::index');
<?php namespace App\Models;
use CodeIgniter\Model;
class MyStudents extends Model
{
protected $DBGroup = 'default';
protected $table = 'students';
protected $primaryKey = 's_id';
protected $returnType = 'array';
protected $useTimestamps = true;
protected $allowedFields = ['s_name','s_date','s_subject','s_update'];
protected $createdField = 's_date';
protected $updatedField = 's_update';
}
Note: For Bootstrap and jquery, because we are using the jquery and Bootstrap files in our views so, add the
bootstrap 4 in Codeigniter 4
You can also add your extra
CSS and JS files
Step 9: Create a view named "newstudent" and keep this view in your view folder available in app/views; copy the below code and paste it into the file you have just created.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<form action="<?php echo site_url('students/updatestudent'); ?>" method="post">
<p>Student Name
<input type="text" name="std" placeholder="Student Name" value="<?php echo $student[0]['s_name']?>">
</p>
<p>Student Subject
<input type="text" name="subject" placeholder="Student Name" value="<?php echo $student[0]['s_subject']?>">
</p>
<input type="hidden" value="<?php echo $student[0]['s_id']?>" name="id">
<button type="submit">Update Student</button>
</form>
</body>
</html>
Step 10: Create a view named "editstudent" and keep this view in your view folder available in app/views; copy the below code and paste it into the file you have just created.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<form action="<?php echo site_url('students/updatestudent'); ?>" method="post">
<p>Student Name
<input type="text" name="std" placeholder="Student Name" value="<?php echo $student[0]['s_name']?>">
</p>
<p>Student Subject
<input type="text" name="subject" placeholder="Student Name" value="<?php echo $student[0]['s_subject']?>">
</p>
<input type="hidden" value="<?php echo $student[0]['s_id']?>" name="id">
<button type="submit">Update Student</button>
</form>
</body>
</html>
Step 11: Finally, create a final view named "students" and keep this view in your views folder; copy the below code and paste it into your file, which you have just created.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="<?php echo base_url('bootstrap/css/bootstrap.css')?>" type="text/css">
<title>Document</title>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<?php if (!empty($message) && isset($message)): ?>
<?php echo $message; ?>
<?php endif; ?>
<br>
<a class="btn btn-success" href="<?php echo site_url('students/newstudent'); ?>">New User</a>
<h1>All Students</h1>
<table class="table">
<th>ID</th>
<th>Name</th>
<th>Subject</th>
<th>Date</th>
<th>Edit</th>
<th>Delete</th>
<?php if(count($students) > 0): ?>
<?php foreach ($students as $myStudent): ?>
<tr>
<td>
<?php echo $myStudent['s_id'];?>
</td>
<td>
<?php echo $myStudent['s_name'];?>
</td>
<td>
<?php echo $myStudent['s_subject'];?>
</td>
<td>
<?php echo $myStudent['s_date'];?>
</td>
<td>
<a class="btn btn-info" href="<?php echo site_url('students/editstudent/'.$myStudent['s_id'])?>">Edit</a>
</td>
<td>
<a class="btn btn-danger" href="<?php echo site_url('students/delete/'.$myStudent['s_id'])?>">Delete</a>
</td>
</tr>
<?php endforeach; ?>
<?php endif; ?>
</table>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script>
<script type="text/javascript" src="<?php echo base_url('bootstrap/js/bootstrap.js')?>"><</script>
</body>
</html>
Step 12: Now go to your browser and access your root folder; in my case, I am going to use this URL http://localhost/crudci4/public/; in the URL, crudci4 is my root or base_url
The complete code also available on Github
You can watch the complete course named Crud Operation In Codeigniter 4
You can also watch the entire Codeigniter 4 series.
https://www.youtube.com/watch?v=C6F3VNpkeuo
0 Comment(s)